Vehicle Input and Attributes
You can control your vehicle directly by modifying the vehicle prim’s attributes
Accelerator and Steering
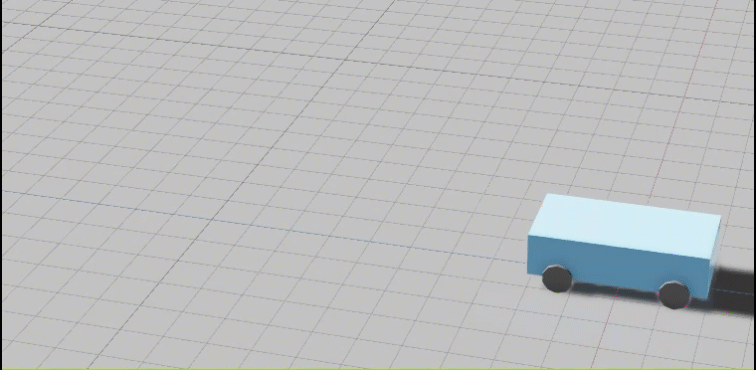
The following code fully applies the accelerator and then, inside the update callback, oscillates the steering from -1 to 1 using the sin function.
import carb
import math
VEHICLE_PRIM_PATH = "/World/WizardVehicle1/Vehicle"
def start_sim(self):
usd_context = omni.usd.get_context()
my_stage = usd_context.get_stage()
self.vehicle_prim = my_stage.GetPrimAtPath(VEHICLE_PRIM_PATH)
update_event_stream = omni.kit.app.get_app().get_update_event_stream()
self._pop_event_stream_sub_id = update_event_stream.create_subscription_to_pop(self.tick_vehicle,name="tick_vehicle_pop")
self.time = 0
self.vehicle_prim.GetAttribute('physxVehicleController:accelerator').Set(1.0)
def tick_vehicle(self, e: carb.events.IEvent):
self.time += e.payload["dt"] * 3.0
new_steer = math.sin(self.time)
self.vehicle_prim.GetAttribute('physxVehicleController:steer').Set(new_steer)
Adding Brakes

The following code, using the same start_sim
fully applies the accelerator, but then after 2.5 seconds releases the accelerator and applies the brakes.
def tick_vehicle(self, e: carb.events.IEvent):
self.time += e.payload["dt"]
if self.time > 2.5:
self.vehicle_prim.GetAttribute('physxVehicleController:accelerator').Set(0)
self.vehicle_prim.GetAttribute('physxVehicleController:brake0').Set(1)